PlatformBoy – Platformer Prototype
For this week, I created a platformer using kenney.nl assets again, just like the top-down shooter. the assets I am using in the game are the following:
The first thing I did with this game was create a mini level and put my character in it so that I will be able to attach scripts and collision to the different areas of the world. I set the Grass and dirt to ground layers, and set the spikes to a layer called damage so that I could implement a damage system into the game like in the space invader and top down shooter prototypes.
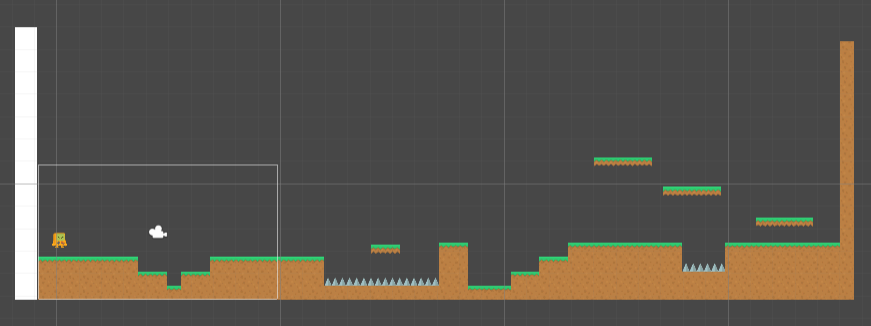
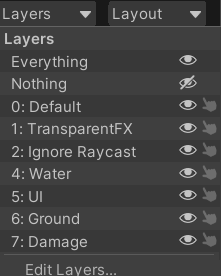
I made a script for the player controls called scr_PlayerBehaviour which controls almost everything in the game, from detecting danger, moving , jumping and more
In the PowerPoint tutorial we read, we were shown how to detect what the character was standing on by using LayerMasks and a child GameObject connected to the player’s feet area. In the PowerPoint this was shown to detect only ground, however, I took the same technique and twisted it slightly to detect when you are on a damage layer too, which I used later in the script to reset my script, respawning the player at the start again.
At the start, the SpriteRenderer component is called, and we use this to flip the sprite depending on the user’s input to make the sprite face the correct way that it’s moving. along with this I also created animations for different movements such as walking and jumping. I didn’t know how to do this initially so I searched up on google to see if I could do it with the sprite renderer by looking through the unity documentation but didn’t find anything about it so instead I found a video on how animate in Unity. I quickly ran into an issue when I couldn’t find the sample size option in the animator window, but that was fixed by finding a different video that showed where it was.
This is what the animator looks like, and how different animations are linked together. There is also an example of the conditions that tell the game how to transition from one state to another.
in the example shown, the animation goes from jumping to idle because he has landed and isn’t moving, making him stand still.
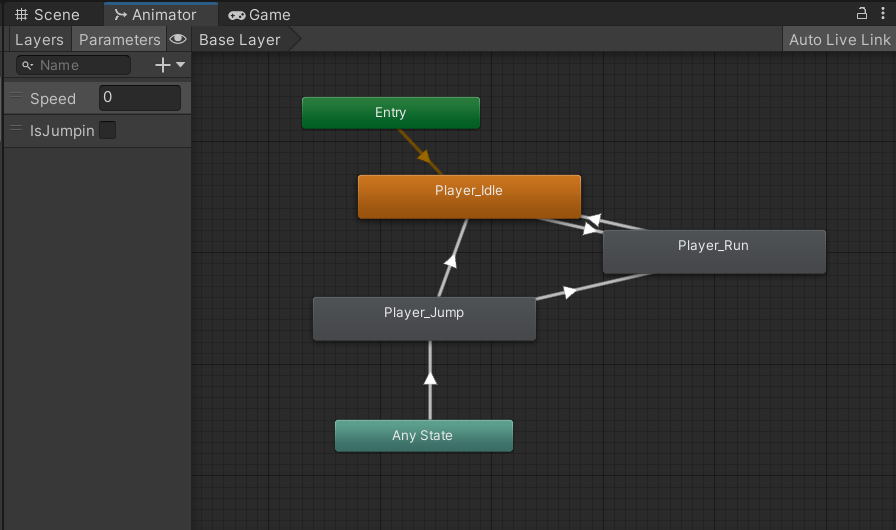
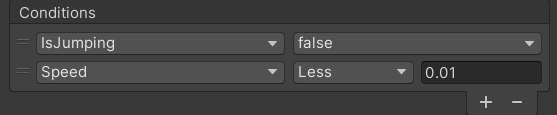
I also wanted to add a way for the camera to follow the character so I added that too, but I needed more research, so I found a Youtube video that walked through it, which lead me to create the script ‘CameraScript’
This script makes the camera focus on the player by clamping to it, but only in a certain range, which I can alter in unity. if the player leaves that range, the camera is unable to go there. This means that we can get close to a wall without letting players see past a certain area such as the border of the level.
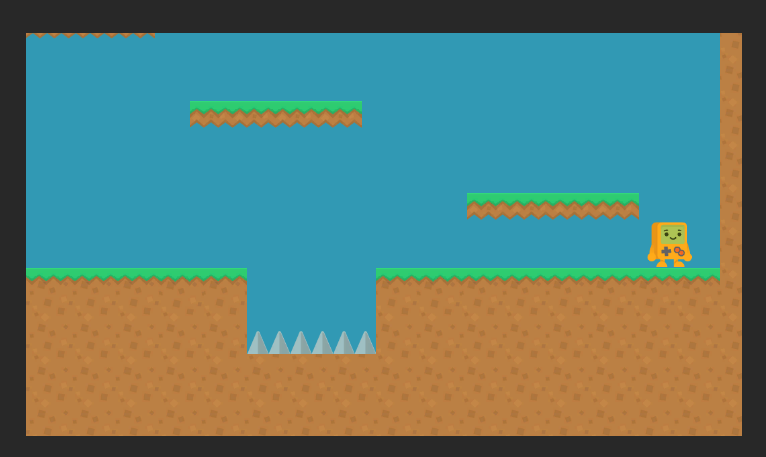
Overall, this is probably the game I’m most proud of so far and had the most fun working on, and the one that got me more used to different unity features such as Mathf.Clamp, Animator, and LayerMasks.
Even though I’m happy with the prototype, if I wanted to make it a proper game I would add more mechanics. I let some of my friends play this and gathered feedback and a common request was more features, such as power-ups, enemies, coins, and an end goal.
Bibliography:
[Accessed 15/11/2021]
Available online https://youtu.be/hkaysu1Z-N8 [Video]
[Accessed 15/11/2021]
Available online https://youtu.be/c5tiMMMcyIA [Video]
[Accessed 15/11/2021]